Hey all,
I recently needed to generate values following a Maxwell distribution. Wikipedia gives the hint that this is a Gamma distribution, which in C++11 is easily useable. Thus, thanks to <random>
, we can setup a Maxwell distribution in a few lines of code:
#include <random>
#include <chrono>
#include <iostream>
using namespace std;
int main()
{
unsigned seed = chrono::system_clock::now().time_since_epoch().count();
default_random_engine generator(seed);
// Boltzmann factor times temperature
const double k_T = 0.1;
// setup the Maxwell distribution, i.e. gamma distribution with alpha = 3/2
gamma_distribution<double> maxwell(3./2., k_T);
// generate Maxwell-distributed values
for (int i = 0; i < 10000; ++i) {
cout << maxwell(generator) << endl;
}
return 0;
}
Pretty neat, I have to say!
continue reading...
Time flies… The extremely productive hack sprint at the friendly Blue Systems office in Barcelona is over for more than a week. I haven’t had time to blog about the last days yet which I hereby make good for!
I spent a lot of time at the sprint polishing the KDevelop Clang plugin. A up-to-now semi-secretly developed language plugin based on clang which will replace our current C++ language support in KDevelop in the long-run.

Code completion ofstd::string
in a std::vector
.

Showing a clang-generated warning inside the editor.
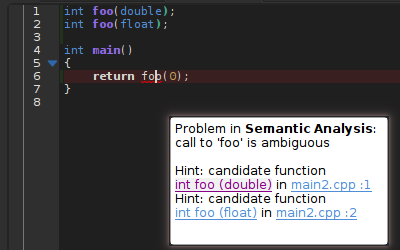
Nested clang diagnostics with browsing support.
KDevelop’s current C++ plugin
But a bit of history first: KDevelop’s current C++ language plugin can pull off what it does by essentially implementing (parts of) the C++ standard. We have our own C++ parser, we have our own implementation of the C++ template mechanism, we have our own share of “compiler bugs”, …. It should not surprise anyone that this is a maintenance nightmare. And indeed, with C++11 and the wealth of changes it came with our language simply fails in many areas. The current codebase is big (~55k sloc) and adding support for complicated features such as variadic templates or constexpr is extremely hard and fragile. So instead of doing that, my colleague Olivier JG started looking into using clang of LLVM fame instead. It promises an API for third-party tools to steer a C/C++/Objective-C compiler to their needs. Its the magic bullet which we waited for, and which did not exist back when KDevelop 4.0 was started initially.
continue reading...
Finally I managed to get my job as the release dude done: <http://kdevelop.org/kdevelop/kdevelop-430-final-released- basic-c11-support>
Thanks to all the developers who sent in patches! The same goes to our loyal users for their continued support and bug reports :)
It’s really fun to work on KDevelop and - I’ve said it many times before - I’m really looking forward to our next releases! Even now our code in the master branches has some neat commits that make the eventual 4.4 release something to look forward to!
continue reading...
Hey all,
I finally want to write a bit about my work on KDevelop during this year’s GSOC. To make things a bit more interesting for the whole crowd, even for those heretics that don’t use KDevelop, I want to highlight some C++2011 features I got to know in the process. Hence this multipart blog post should be interesting for all KDE hackers, as eventually we will be able to use these shiny new features of what is to be known as C++2011.
For those interested in the full overview of changes in C++2011, take a look at e.g. the C++0x Status Page in GCC 4.6, the wikipedia article on C++0x, or try to get hold of a copy of the C++2011 FDIS spec file. Note that the latter is apparently not freely accessible, see also this stackoverflow discussion. Still, maybe you find someone who can send you a copy…
So, lets get down to business. Following is a not-complete list of C++2011 features I’ve already implemented in KDevelop. If I mess something up in explaining a new feature, or if I forget an important aspect, please add a comment.
continue reading...
Yawn. Hello everyone!
After two nights of backporting sleep in my RL branch to fix the deficiency I built up during Randa, I feel somewhat normal again. Time to blog, eh?
Randa
Lets begin with Randa. It was not only my first time there, but also my first time ever to be in the Swiss Alps. And furthermore it was the first time for me to be in the Alps without crying out loud about the lack of snow as I didn’t intend to go snowboarding. You can imagine it was a very pleasant experience for me. I definitely want to come back to go on some more serious hiking trips uphill. But I diverge ;-)
Randa as place for a sprint was simply awesome: Secluded hence no real distractions. Great environment to clean your head, get fresh air and stay focused and productive. Good food, nice people, … I could go on here :) The only negative things I noticed where the unstable networking and the dormitories.
KDevelop Sprint
The KDevelop sprint at Randa was very successful and productive. Here is a group picture of those who attended:
continue reading...
Ok, just a quick update on what we are doing / have done the last two days except drinking beer and eating quite a lot of food (though it never seems to be enough somehow….)
- Apol was polishing CMake support, speeding up KDevelop startup time when you enabled the QtHelp plugin, making sure that Kross plugins get loaded properly and some other things
- Adymo started some refactoring of Sublime in an experimental branch (actually they had quite a long discussion right now about things in the UI which should be changed/fixed). Besides that he also fixed some little bugs in the includepath resolver for CMake
- Nsams is fixing PHP support and adding new features here and there. Esp. completion inside a foreach over a Iterator class works now!
- and as always ZWabel did fix quite a lot of bugs, most of them which only were discoverable in a few corner cases, very nice indeed.
- shaforo who sadly already left us again to attend university fixed the sorting in the Project view among other things
- With Apols help I managed to get started with a documentation plugin which (currently) integrates PHP.net. I plan to make it possible to use the downloadable PHP.net documentation as well to speed things up. Actually setting up a documentation plugin is very easy! Maybe I’ll take a look at writing a documentation plugin for Zend, Symfony, etc. pp. as well - should be fairly easy. Maybe I can come up with a generic documentation plugin which makes it possible to include any kind of
.html
documentation with some configurable mapping… lets see! Or maybe I should take a look at writing Kross plugins (Apol could help me here as well) for that purpose.
continue reading...
Hello Planet KDE!
I want to give you a little insight on the current state of PHP support in KDevelop4:
Me and Nikolaus Sams (nsams) are working diligently on a plugin for PHP support in playground. It’s somewhat stable, i.e. we fix any crashes we stumble upon, but I would call it Alpha state at most. It may eat your babies so to speak. Yet I’m happy to say that at least one user is already using it for production (hi leinir ;-) ).
implemented PHP support
Well, here’s a (not complete) list of features that are already working. Though I have to warn you: no screenshots included ;-) It’s actually all very similar to the C++ screenies you can see on the web.
sematic highlighting
Let’s start with a feature that only very recently was added for PHP - semantic highlighting. Niko moved some language independent parts of the C++ plugin from KDevelop to KDevplatform and now PHP has the same code highlighting features as C++.
continue reading...
Kirocker is such a great application, it’s a pity the developer Sébastien Laoût has given up on it and is using Windows now!
Usually I only use the kicker applet, the full screen window is nice for parties though. But I didn’t like the way the applet handles scroll events: It seeks (if possible). Since I rarely seek when listening to music I’d rather have the behaviour of the Amarok tray icon: volume control!
I had a look inside the sources and found this part in src/coverdisplay.cpp
:
void CoverDisplay::wheelEvent(QWheelEvent *event)
{
if (areControlsShown()) {
if (event->orientation() == Qt::Vertical) {
PlayerInformation *infos = PlayerInformation::instance();
if (infos->canSeek()) {
int deltaSeconds = 10 * (event->delta() > 0 ? 1 : -1);
m_infos->seekRelative(deltaSeconds);
}
} else {
if (event->delta() > 0)
AmarokApi::volumeUp();
else
AmarokApi::volumeDown();
}
}
}
continue reading...